Loops in Java
Loop in programming concept is block of code which provides a set of instruction that keeps executing until a specific condition is met and becomes true/false to stop its execution,whether execution needs to be stopped on true or false depends on the condition that is implemented to stop its execution. In Java there are three ways to execute a loop which as follows
1) while loop
2) for loop
3) do while loop
while loop :
A while loop is a control flow statement in which a set of instructions is being executed repeatedly based on a given Boolean condition
while loop syntax :
while (boolean condition)
{
// Execute a set of statements
// increment
}
Program : Java while loop Program
public class whileLoopProgram {
// Java while loop Program
public static void main (String[] args) {
int i=0;
while (i<=12)
{
System.out.println(i);
i++;
}
}
}
Output :
0
1
2
3
4
5
6
7
8
9
10
11
12
Flowchart of while loop
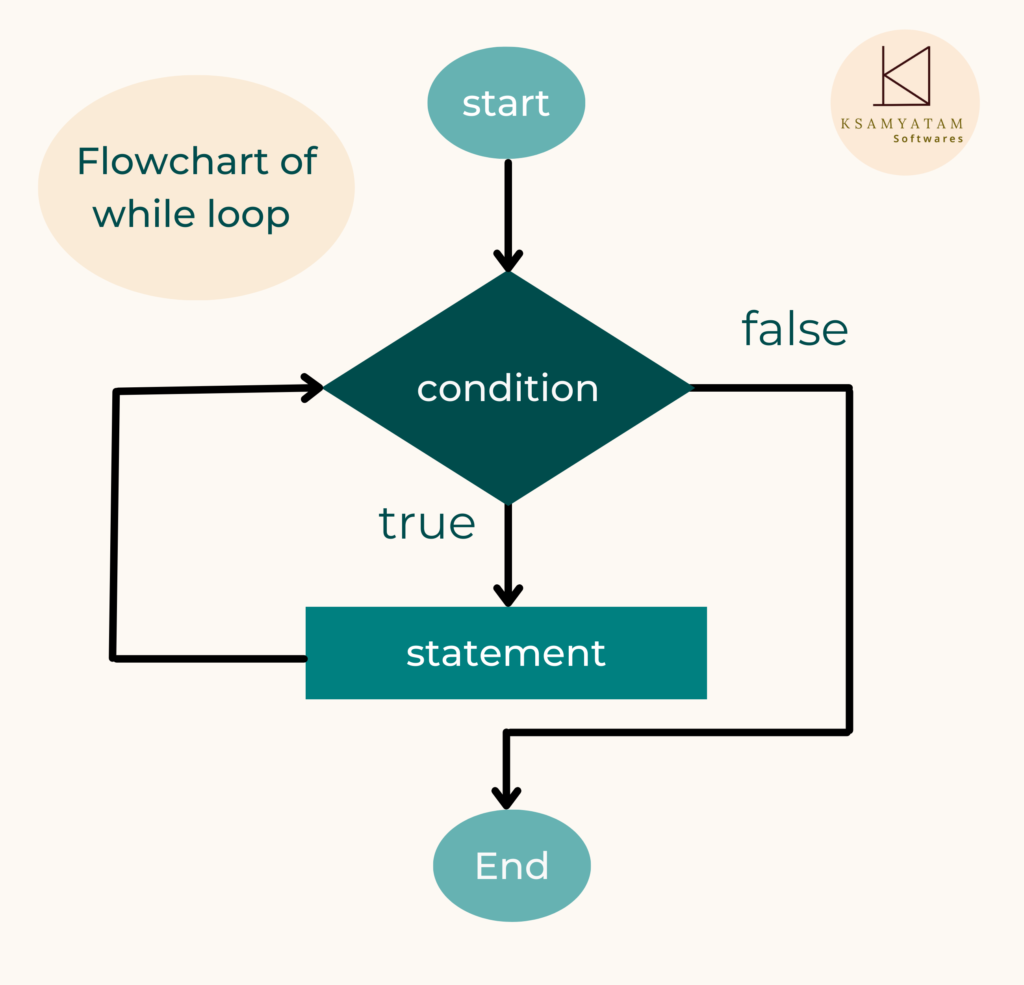
for loop :
for is a control structure which keeps executing block of code for specific number of times, which are called iterations, until condition is met. for loop uses initialization, condition and increment/decrement to execute block of code repeatedly. There are various types of for loop which are empty for loop, infinite for loop, and foreach loop.
Initialization: It is used to initialize a counter variable.
Condition: Condition is used to keep loop running as long as this condition is true.
Increment/Decrement: Here the counter variable is incremented or decremented to execute the next iteration.
for loop syntax :
for (initialization; Condtition;increment/decrement)
{
// code block to be executed repeatedly
}
Program : Java for loop Program
public class forLoopProgram {
// Java for loop Program
public static void main (String[] args) {
for (int i=0;i<=12;i++)
{
System.out.println(i);
}
}
}
Output :
0
1
2
3
4
5
6
7
8
9
10
11
12
Flowchart of for loop
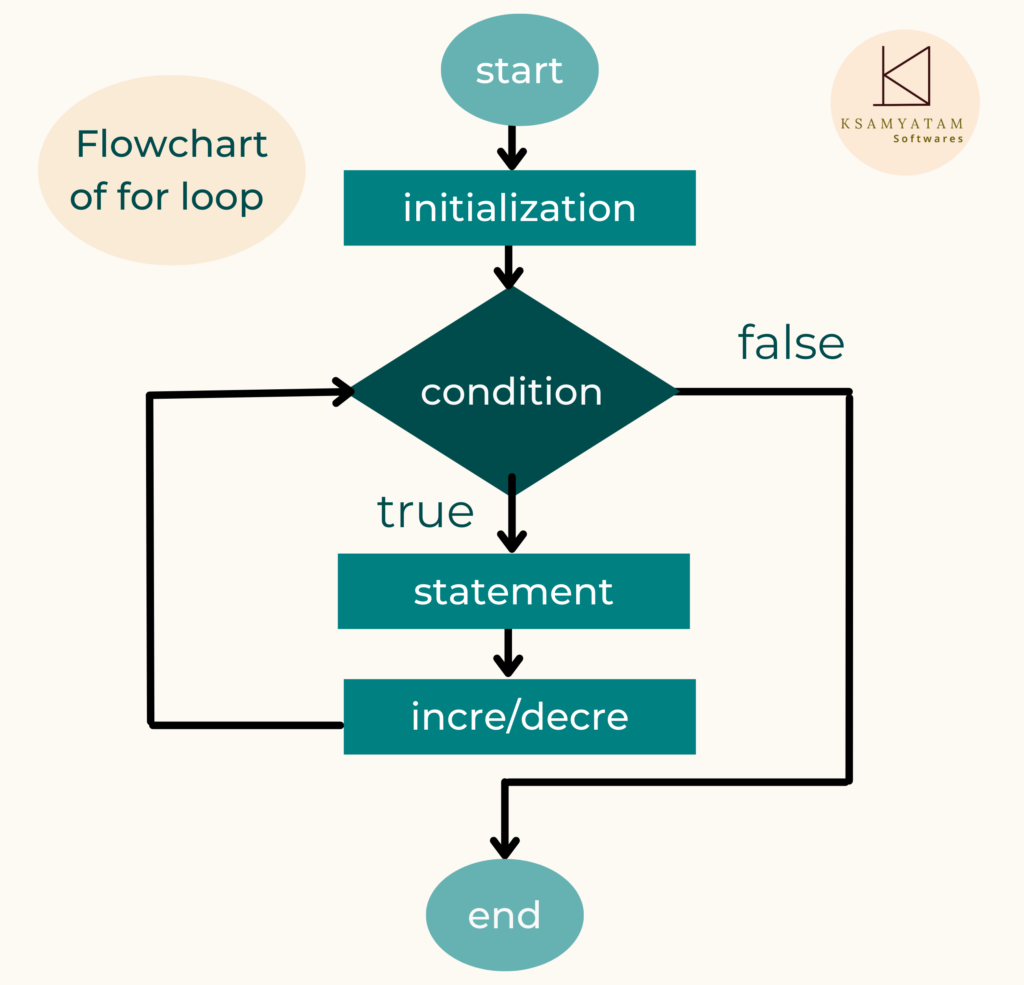
do while loop :
do while loop is similar to while loop with only difference that it checks for condition after executing the statements, and so it’s a Exit Control Loop.
do while loop syntax
do
{
//code to be executed
//update statement
}
while (condition);
Program : Java do while loop Program
public class doWhileLoopProgram {
// Java do while loop Program
public static void main (String[] args) {
int i=0;
do
{
System.out.println(i);
i++;
}
while(i<=12);
}
}
Output :
0
1
2
3
4
5
6
7
8
9
10
11
12
Flowchart of do while loop
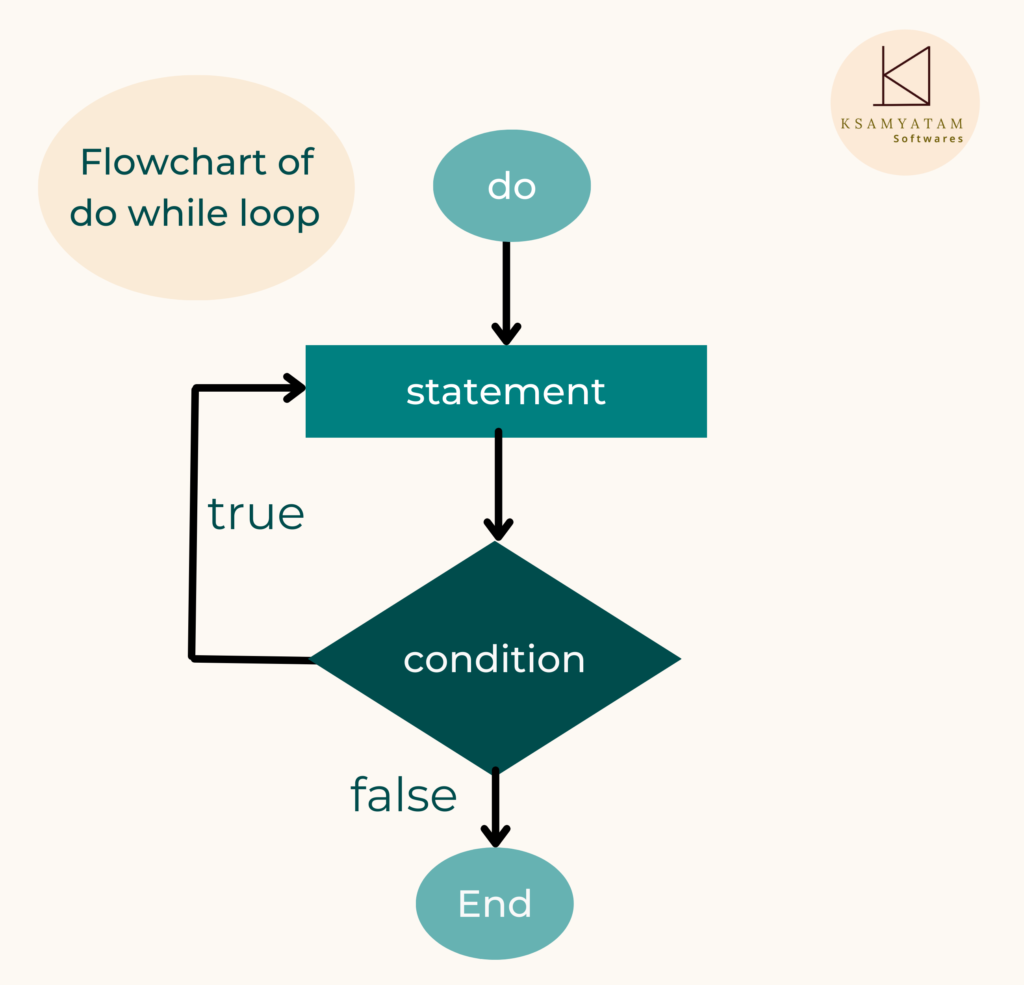