Jump Statements
Jump statements are control statements that transfer execution control from one point to another point in the program,allowing for changes in the flow of execution. There are three Jump statements that are provided in the Java programming language:
1) Break Statement
2) Continue Statement
3) Return Statement
1) Break Statement :
A break statement in Java is used in terminating the execution of a loop in a particular program.It transfers the control of the program to the next statement which is immediately after the current loop or switch.There are 3 types of Break Statements, those are “Exiting a Loop”, “As a form of Goto”, and “In a switch case”.
i) Using Break Statement to exit a loop:
It is generally used for getting exits from any existing loop.When a break statement is found inside a loop, the loop is terminated, and the control reaches the statement that follows the loop.
Syntax:
break;
Program : Java Program For Break Statement to exit a loop
public class breakExitLoopProgram {
public static void main(String[] args)
{
int n = 12;
for (int i = 0; i < n; i++) {
if (i == 5)
break;
System.out.println(i);
}
}
}
Output :
0
1
2
3
4
ii) Use Break as a form of goto
Java does not have a goto statement but it provides a “Break label” statement for executing a particular label block.Labeled break statement is used to terminate the outer loop, the loop should be labeled for it to work.This form of break works with the label. The label is the name of a label that identifies a statement or a block of code.
Syntax:
break label;
Program : Java Program For Break Statement as a form of goto (Break label)
public class breakLabelProgram {
public static void main(String[] args)
{
for (int i = 0; i < 6; i++) {
A : { // label one
B : { // label two
C : { // label three
System.out.println("Value of i=" + i);
if (i == 0)
break A; // break to label one
if (i == 3)
break B; // break to label two
if (i == 4)
break C; // break to label three
} // label three ends
System.out.println("After label C");
} // label two ends
System.out.println("After label B");
} // label one ends
System.out.println("After label A");
}
}
}
Output :
Value of i=0
After label A
Value of i=1
After label C
After label B
After label A
Value of i=2
After label C
After label B
After label A
Value of i=3
After label B
After label A
Value of i=4
After label C
After label B
After label A
Value of i=5
After label C
After label B
After label A
iii) Using Break Statement in a Switch case:
In Java when a switch case program reaches a break keyword, it breaks out of the switch bloc and stop the execution of more code and case testing inside the block.
Syntax :
switch (expression)
{
case value1:
statement1; // Execution of statement 1 if case is value 1
break;
case value2:
statement2; // Execution of statement 2 if case is value 2
break;
.
.
case valueN:
statementN; // Execution of statement N if case is value N
break;
default:
dfaultStatement; // Execution of default statement if none of above value gets executed
}
Program : Java Program Using Break Statement in a Switch case
public class breakSwitchCaseProgram {
public static void main(String[] args)
{
char a = 'G';
switch (a)
{
case 'B':
System.out.println("Letter B Found");
break;
case 'G':
System.out.println("Letter G Found");
break;
default:
System.out.println("Default case: NO Letter Matched!");
}
}
}
Output :
Letter G Found
Flowchart break statement
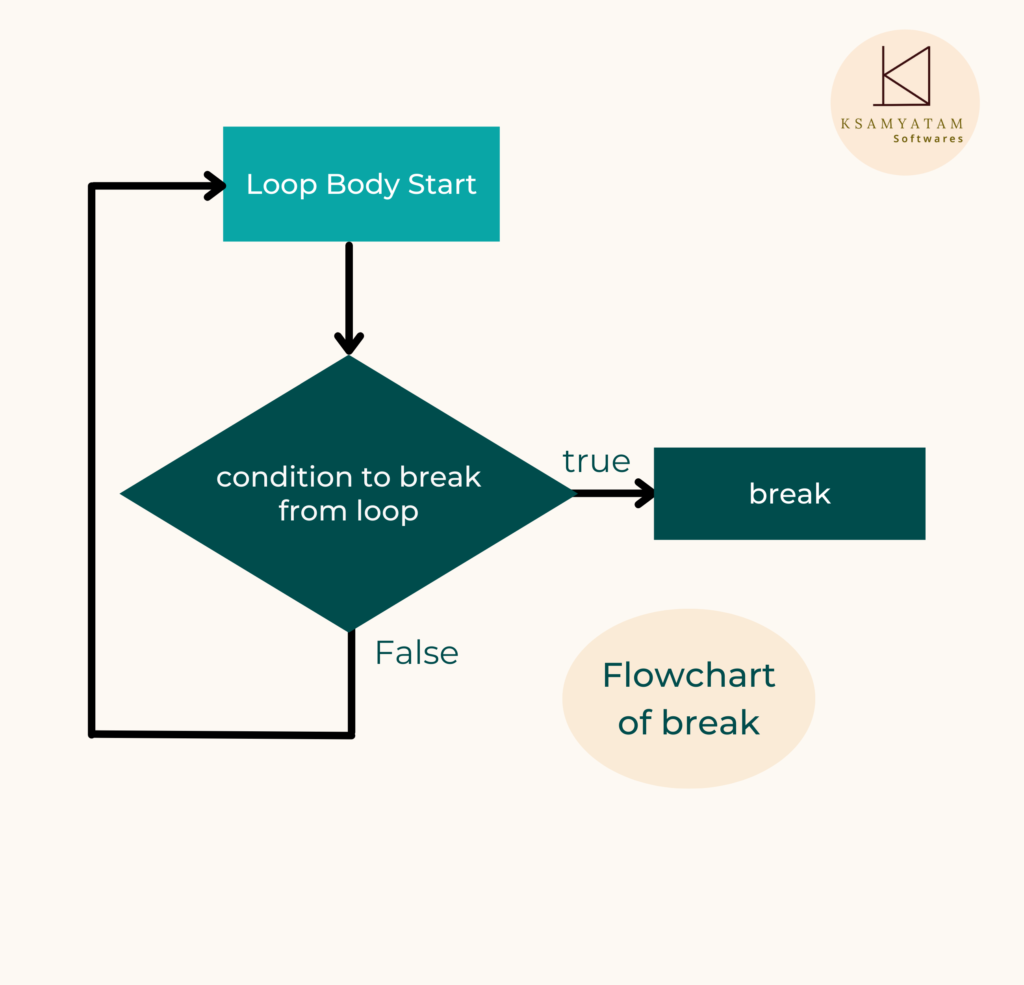
2) Continue Statement :
In Java the continue statement never terminates the execution of any loops. It can only work inside the loop statement.The primary job of the continue statement is the iteration of that specific loop.continue statement assists the bypass of all the other statements by making them fall under it.The job of the continue statement is to skip the current iteration and force for the next one.
Syntax :
continue;
Program : Java Program Using continue Statement
public class continuePorgram {
public static void main(String[] args)
{
for (int i = 0; i < 12; i++) {
if (i == 4)
{
continue;
}
System.out.println(i);
}
}
}
Output :
0
1
2
3
5
6
7
8
9
10
11
Flowchart continue statement
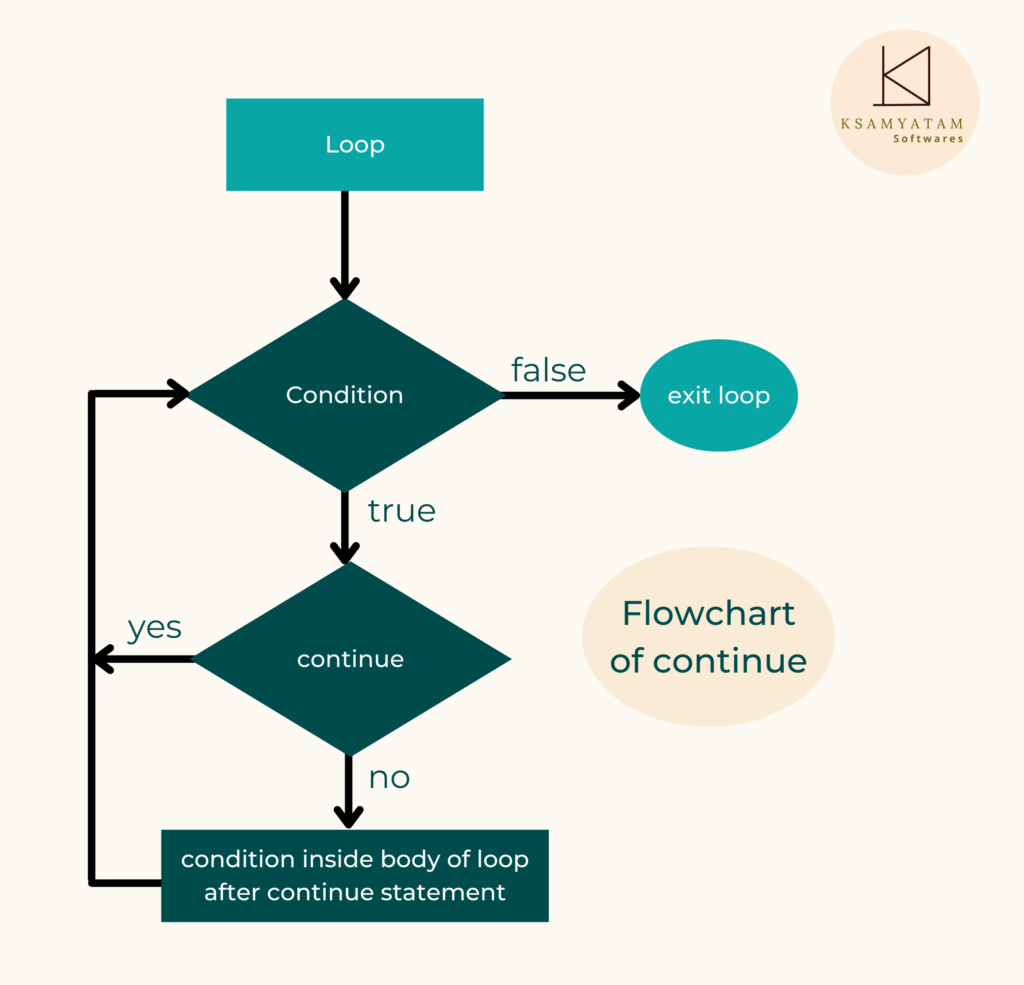
3) Return Statement :
The return statement in Java is a type of jump statement which is primarily used only inside the functions or methods and any code that is written after using the return statement is treated as an unreachable statement by the compiler.The purpose of using a return statement is to terminate the current method of execution and transfer the control to the next “calling method”.Since the control jumps from one part of the program to another, the return is also a jump statement.“return” is a reserved keyword means we can’t use it as an identifier.There are two types of Return statements, which are “Return with a value” and “Return without a value”.
Syntax :
return;
Program : Java Program Using return Statement
public class returnProgram {
public static int calculateMultiply(int num1, int num2)
{
System.out.println("Calculating the Multiplication of " + num1 + " and " + num2);
int result_num = num1 * num2;
System.out.println("The Multiplication is: " + result_num);
return result_num;
}
public static void main(String[] args)
{
int result = calculateMultiply(2, 5);
System.out.println("Result: " + result);
}
}
Output :
Calculating the Multiplication of 2 and 5
The Multiplication is: 10
Result: 10